Model In Laravel -: We know that the laravel is a php framework. Laravel work on MVC pattern.When we create any application or web page then we create a database. In the laravel framework for connecting database there are two way. First is the model and second is the normal database classes. In other words we can say that a model is a class that show a logical structure between the database table.
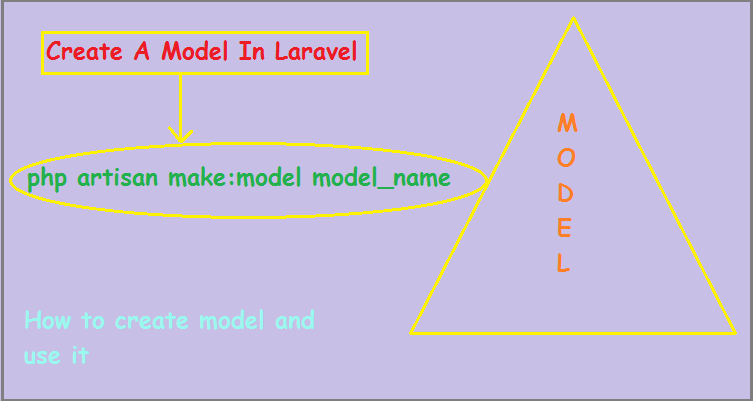
Database table’s are map with the laravel class . For example if you have a table in database and table name is the users then you create a model. Model name is the user. Then it’s understand automatic and map users table with the user model.
Model is created in singular form and database table is created in plural form. If we are creating a table dive_details then we create the dive_detail model in laravel.
How to Create Model In Laravel
We Know that laravel is a command line language. All the controller and model are creating by the command. For Creating a Model we use the command. In laravel 7 and its below version model’s are available in the app folder. Now the laravel 8 and it’s higher version model are show in the Model folder that is available in the app folder.
For creating a model first we open the project using the cmd command. Now we give the command for creating Model
php artisan make:model model_name
Here we give the model name here if we have a users table in database then we give this command in laravel
php artisan make: model user
User model has been created in the model folder. Now we open the model folder and open the user model. It will show here as like
<?php
Namespace App\Model;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class user extend model
{
use HasFactory;
}
How to access model in laravel-: We can use model with the controller. For accessing any model we create a controller and then we use it. After creating a controller we can define this model in the controller. Here we will create a user_controller and a function that name is user_data.
<php ?
Namespace App\http\controllers;
use illuminate\http\Request;
use App\Models\user; // for accessing the model
Class user_controller extend controller
{
public function user_data() // user_data is a function name
{
return users::all(); //here users is a table name and its return all data.
}
}
After creating a controller we make a route in laravel. In the web.php file we create a route of this controller as like
Route::get(‘users’,[user_controller::class,’user_data’];
Now run this program all the users table data will show here.
What is Migration In Laravel
With the migration you can generate database table with the code. You don’t need to create a database table using the database. Using the migration we can create a table in the database. You don’t need to crate table manually.
How to Create a Migration In laravel-: In the laravel we can give a simple command and create a migration in laravel. For creating a migration we open the project using the cmd command and give this command
php artisan make:migration create_table-name_table
here table-name is the database table name. for example we give the test table name. You can change it according you. Your migration has been created. For seeing your migration go to the database folder here a migration folder is available open it. Here you will see two function up and down . Its looks like this way
<php ?
use illuminate\Database\Migrations\Migration;
use illuminate\Database\Schema\Blueprint;
use illuminate\Database\Support\Facades\Schema;
Class classtest extend migration
{
public function up() // It will use for creating a new table
{
Schema::create('test',function (Blueprint $table) {
$table->id(); // this are by default
$table->string('name');
$table -> string('father_Name');
$table->timestamps(); // this are by default
}
}
public function down()
{
schema::dropIfExists('test); // this is use for delete table
}
}
When we create a migration then two function will automatic created. First is the up function there are two field are available. these are the id field and timestamps. here we can add another field name as like name or father name etc. up function is use for creating table and its field name.
Another function is the down function. Down function will use for delete the table.
How to create a table field in database using the laravel –: For creating a table field name we give the field name in the migration up function and save it. After saving we use this command
php artisan migrate
when we give this command all the table has been created in the database.