Introduction of API-: Application Programming Interface (API) is the standard format of API. It is a basic terms, APIs just allow application to communicate with one another. An API is the messenger that delivers your request to the provider that you are requesting it from and then delivers the response back to you. This is not a database. It is an access point to an app that can access a database.
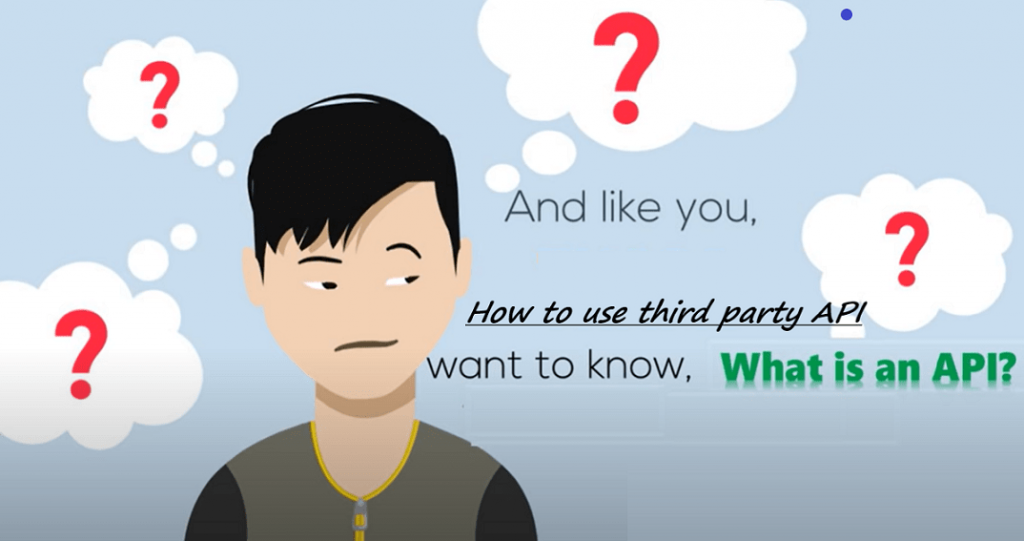
How to use third party API with jQuery
We create a project here for using third party API. Here I am using subline text editor for creating a project you can select a notepad or any other app. Follow these process for creating a project.
1-: First create a folder and give a name of folder here I am giving the name of folder test_api. Open this folder and create a file and give the name of file Index.html.
2-: In the test_api folder create another file and give the file name style.css. Here we write the style code here.
3-:Now open the index.html file and write the code here as like show here. Here we will give the button and we use API on this button for changing image .You can download here
<html>
<head>
<title>Use third party api</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<section>
<h1>Your name write here </h1>
<div class="centerDiv">
<div class="details">
<label>ENTER YOUR NAME</label><br>
<input type="text" name="" id="netnicid">
</div>
<div class="imgdiv">
<img src="img.png" width="200" height="200" class="imgchange">
</div>
<button> Click Me</button>
</div>
</section>
</body>
</html>
Here we will create a simple html page now and we will use API in this page. For changing the user interface we use style sheet and giving the name of this style.
4-: Now open the style.css page and write the code here as I am using here.
*{padding: 0; margin: 0; box-sizing: border-box; font-family: 'Luckiest Guy', cursive;}
section{width: 100%; height: 100vh; display: flex; justify-content: center; flex-direction: column; align-items: center;background: #d22ed5}
section h1{ text-shadow: 2px 5px 5px #2f3542; word-spacing: 10px; margin-bottom: 15px;
font-size:2.5rem; text-transform: uppercase; letter-spacing: 2px; color: white;}
.centerDiv{ width: 60%; height: 450px; box-shadow:2px 2px 2px 5px white; display: flex;
flex-direction: column; align-items: center; justify-content: center; background: #57606f; color: white;}
.details{ text-align: center; text-transform: uppercase; }
.details label{font-size: 1.4rem;}
.details input { padding: 10px; background-color: #ff6b81; color: white; text-align: center;}
.details input[type=text]:focus {
border: 3px solid #555;
}
.imgdiv {margin: 30px 0;}
button {
background: #3498db;
width: 180px;
padding: 4px 0;
border-radius: 3px;
color:white;
font-size: 1.2rem;
letter-spacing: 2px;
background-color: darken(#f1c40f, 20%);
}
button:hover {
cursor: pointer;
}
This is the common thing for creating a index page.
In this folder we given a image that’s name of img.png.
5-: How to add jQuery in Index page
Now next steps comes for adding jQuery. For adding jQuery you can write this code direct or you can go to the google and search jQuery.
Here you can find the jQuery code like here or you can copy this code and paste in your index page before closing the body tag.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js">
</script>
6.-: How to use Third Party API
Using third party API we want to add this API link on our page. Some API giving free of cost and some are taking money. Here I am using free API for changing image. I take this website API for joeschmoe.io
This website provide the API for free of cost. I am using here also this website API you can also use this website API
https://joeschmoe.io/api/v1/
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('button').click(function(){
const netnic = $('#netnicid').val();
alert(netnic);
$('.imgchange').attr('src',`https://joeschmoe.io/api/v1/${netnic}`);
const b = $('.imgchange');
console.log(b);
});
});
</script>
In this we start jQuery code with <script> function. Now we start to write jQuery code with $ Sign. we know that the $ sign is a definition of jQuery. Next we write a function code here as like $(document).ready(function() {
In the button we want to function will start and show the alert. then we write a simple alert function as like $(‘button’).click(function()){ Here button is a tag element and we want to some one click one this button then the alert show.
Now we declare a const name is netnic here we store value on netnic variable. It will store input field value. In the input field value has a id ‘netnicid’. Id will declare using the # symbol as like #nentnicid.
Here we give the another const for changing the image. In this variable we pass the third party API. First we take the imgchange class name that was given the class name of image. Now we write this code
$(.imgchange).attr(‘src’,https://joeschmoe.io/api/v1/${netnic}
);
here .imagchange is the class name and attr is the attributes. We want to change src name here that are coming from this API.
Now the save this program and run this will show as like as here
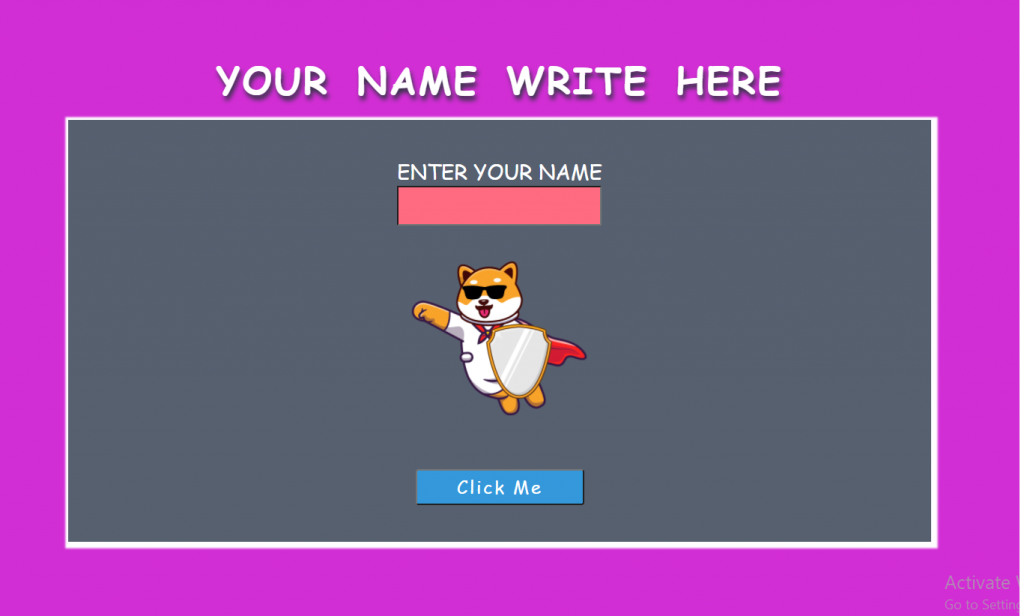
When we give the name and click the button then the image will changes. When we give the same name then we get the same image because it store the value.